Привет всем!
Сел сейчас разбираться с сокетами, столкнулся с проблемой: как принять данные до их конца? Я, например, не врубился :(, и вынужден просить помощи на форуме. Пожалуйста помогите!
-----
/*
КЛИЕНТ
*/
#include <string.h>
#include <netdb.h>
#include <unistd.h>
#include <sys/poll.h>
#include <fcntl.h>
using namespace std;
const char *addr = "localhost";
const int port = 15908;
typedef void* ptr;
typedef struct
{
char ver;
char type;
int len;
ptr data;
} our_packet;
int main()
{
struct sockaddr_in name;
struct timeval timeout;
struct hostent *hostinfo = NULL;
if (!(hostinfo = gethostbyname(addr)))
{
cout << "fuck! gethostbyname failed! :(" << endl;
return 1;
}
int sock = socket(PF_INET, SOCK_STREAM, 0);
name.sin_addr = *((struct in_addr *)hostinfo -> h_addr);
name.sin_port = htons(port);
name.sin_family = AF_INET;
if (connect(sock, (sockaddr *)&name, sizeof(struct sockaddr_in)) == -1)
{
cout << "fuck! connect failed! :(" << endl;
return 2;
}
our_packet pkg;
pkg.ver = 1;
pkg.type = 2;
pkg.data = (ptr)"test\0";
pkg.len = strlen((char*)(pkg.data));
ptr tosend = &pkg;
int len = sizeof(pkg);
int total = 0, n = 0;
while (total < n)
{
if ((n = send(sock, (char*)tosend + total, len - total, 0)) == -1)
{
cout << "fuck! send failed! :(" << endl;
return 3;
}
total += n;
}
cout << total << " of " << len << "bytes sent..." << endl;
char buf[1024];
if (recv(sock, buf, 1024, 0) <= 0)
{
cout << "fuck! recv failed! :(" << endl;
return 4;
}
close(sock);
if (strcmp(buf, "ok") == 0)
{
cout << "all done!" << endl;
return 0;
} else {
cout << "fuck off %)" << endl;
return 5;
}
}
-----
/*
СЕРВЕР
*/
#include <iostream>
#include <string.h>
#include <netinet/in.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <sys/time.h>
using namespace std;
const int port = 15908;
typedef void* ptr;
typedef struct
{
char ver;
char type;
int len;
ptr data;
} our_packet;
int main()
{
int sock = socket(PF_INET, SOCK_STREAM, 0);
struct sockaddr_in addr;
addr.sin_addr.s_addr = htonl(INADDR_ANY);
addr.sin_port = htons(port);
addr.sin_family = AF_INET;
if (bind(sock, (struct sockaddr *)&addr, sizeof(addr)) != 0)
{
cout << "fuck! bind failed! :(" << endl;
return 1;
}
if (listen(sock, 2) != 0)
{
cout << "fuck! listen failed! :(" << endl;
return 2;
}
int nsock = accept(sock, NULL, NULL);
if (nsock == -1)
{
cout << "fuck! accept failed! :(" << endl;
return 3;
}
char buf[1024];
if (recv(nsock, buf, 1024, 0) <= 0)
{
cout << "fuck! recv failed! :(" << endl;
return 4;
}
// что делать с принятыми данными, чтобы сформировать структуру типа our_packet точно такую же, как и у клиента?
sprintf(buf, "ok");
if (send(nsock, buf, 1024, 0) <= 0)
{
cout << "fuck! send failed! :(" << endl;
return 5;
}
close(sock);
return 0;
}
----
PS: что-то в мыслях у меня туманно :(
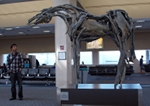
Ответ на:
комментарий
от Spectr

Ответ на:
комментарий
от anonymous

Ответ на:
комментарий
от anonymous

Ответ на:
комментарий
от KIV

Ответ на:
комментарий
от EViL

Ответ на:
комментарий
от anonymous

Ответ на:
комментарий
от EViL
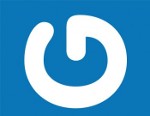
Ответ на:
комментарий
от cvv

Ответ на:
комментарий
от EViL
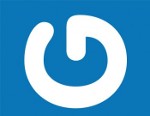
Ответ на:
комментарий
от cvv

Ответ на:
комментарий
от EViL

Ответ на:
комментарий
от anonymous

Ответ на:
комментарий
от anonymous

Вы не можете добавлять комментарии в эту тему. Тема перемещена в архив.
Похожие темы
- Форум Подключиться к unix-сокету через bash (2020)
- Форум Таймаут для connect() (2009)
- Форум Сокеты в Си (продолжене) (2005)
- Форум Не понимаю почему не работает (2013)
- Форум Сокеты передача сообщений между компьютерами. Помогите найти ошибку. (2012)
- Форум [C] Получить данные на TCP сокет (2008)
- Форум Проблема повторного соеденения клиента к серверу через сокет (C++) (2015)
- Форум Работа с сокетами в C (2010)
- Форум Клиент, сервер и их правильная работа. (2005)
- Форум многопоточное копирование фала через сокет (2015)